What is Selenium?
Selenium is a tool for testing web applications. Selenium tests run directly on browsers. Supported browsers include IE (7, 8, 9, 10, 11), Mozilla, Firefox, Safari, Google Chrome, opera, edge, etc. The main functions of this tool include: testing compatibility with browsers - and testing applications to see if they can work well on different browsers and operating systems. Test system functions -create regression tests to verify software functions and user requirements. Support automatic action recording and automatic generation of Net, Java, Perl and other test scripts in different languages.
What is a Selenium Script
A Selenium script is instructions written to automate interactions with a web browser. It simulates user actions such as clicking links, entering text, and navigating between pages. These scripts are essential for testing web applications and ensuring they function correctly across different browsers and platforms.
Selenium, an open-source framework, provides tools like WebDriver to facilitate browser automation. Developers and testers can write Selenium scripts in various programming languages, including Java, Python, C#, Ruby, and JavaScript, allowing flexibility based on the project's requirements. By executing these scripts, teams can perform repetitive testing tasks efficiently, identify bugs, and verify that web applications meet specified requirements.
For example, a Selenium script can automate logging into a website by locating the username and password fields, entering credentials, and clicking the login button.
What are the advantages of making Selenium so widely used?
JavaScript is used by the bottom layer of the framework to simulate real users to operate the browser. The browser will automatically click, input, open, verify and perform other operations according to the script code when the test script is executed. Like what real users do, test the application from the end-user's perspective, enabling automation of browser compatibility testing, although there are subtle differences between different browsers.
Easy to use, you can use Java, Python, and other languages to write use case scripts.
- Selenium is completely an open-source framework and has no restrictions on commercial users. It supports distribution and has mature community and learning documents
- Selenium tests can be run on Internet Explorer, Chrome, and Firefox on Windows, Linux, and Macintosh. No other testing tool can cover so many platforms. There are many other benefits to using Selenium and running tests in browsers.
- Easy to use, you can use Python, Java, Perl, PHP, Ruby and C#, and other languages to write use case scripts.
- Selenium test environments can be built in Windows, Mac, and Linux.
- To achieve CI/CD, Selenium can be integrated with Maven, Jenkins, and Docker to achieve CI/CD.
- It can also integrate with tools like JUnit and TestNG for test management.
- By writing Selenium test scripts that imitate user operations, you can test applications from the end-user's perspective.
- It is easier to find browser incompatibility by running tests in different browsers. Selenium's core, also known as browser BOT, is written in JavaScript. This allows test scripts to run in supported browsers. The browser BOT is responsible for executing the commands received from the test script, which is either written in HTML table layout or a supported programming language.
How to Write Test Scripts in Selenium?
To build up your Selenium test environment, you may want to visit https://www.selenium.dev/downloads/
How you build and configure WebDriver depends on what programming language environment you want to use with your tests. The most popular OS has a package out-of-the-box for installation.
Basics of Selenium Installation
Selenium Suite of Tools:
- Selenium RC(Deprecated from Selenium V3 onwards)
- Selenium IDE
- Selenium Grid
- Selenium WebDriver.
We'll skip the introduction of Selenium RC as it is already outdated. We'll introduce the rest three tools as follows:
Selenium IDE (Integrated Development Environment)
If you want to create issue reproduction scripts to help automate assisted exploratory testing, your first choice would be Selenium IDE, with which Chrome, Firefox, and edge plug-ins can simply record and playback the browser interactions.
The advantage of Selenium IDE is that tests recorded through plug-ins can be exported in various programming languages: Java, ruby, python, etc.
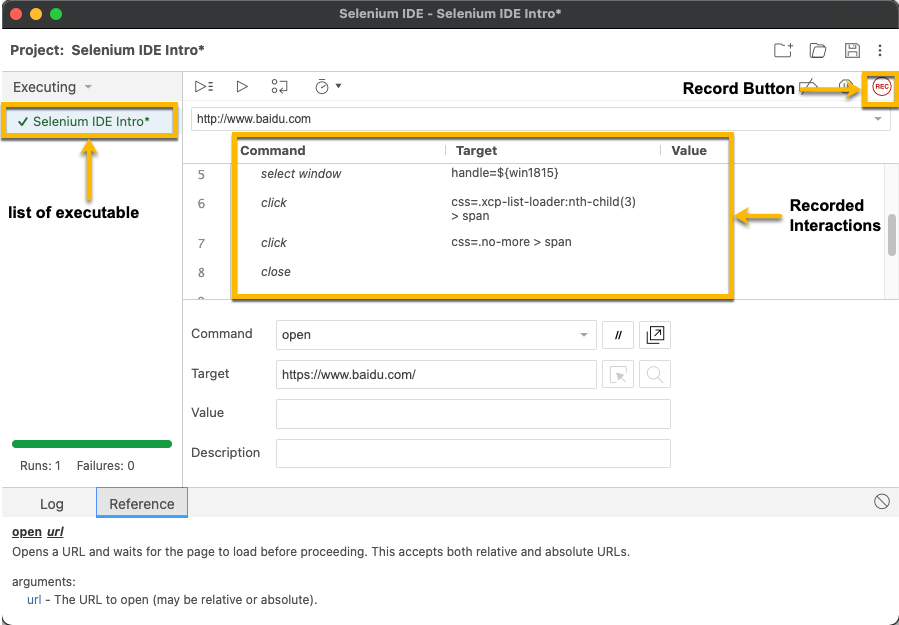
Selenium Grid
If you need to quickly distribute and run tests on multiple machines to extend and manage multiple environments to run tests against various browsers / operating systems, you need to use a Selenium grid.
Several online platforms provide the online Selenium grid, which you can access to run your Selenium automation scripts. You may want to
https://www.selenium.dev/downloads/
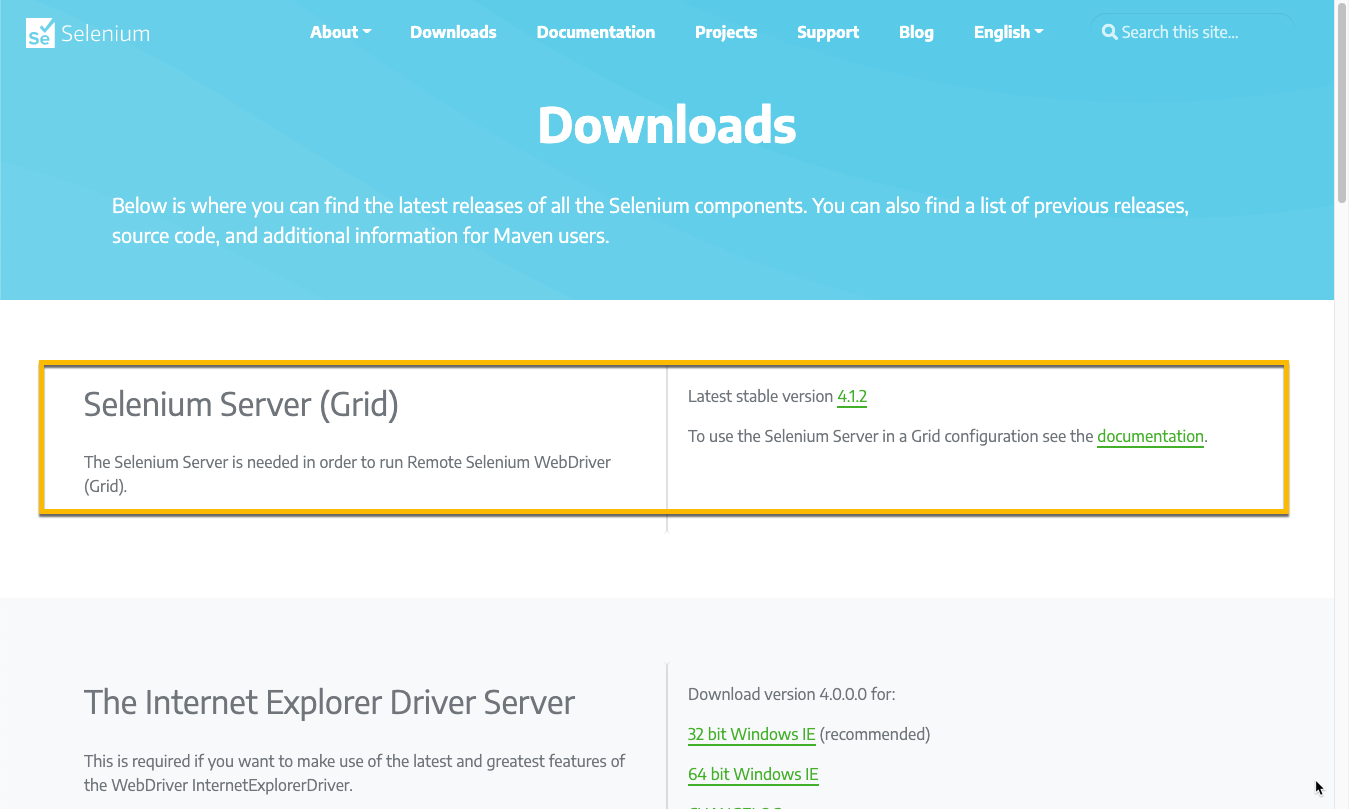
Selenium WebDriver
This enables you to create robust, browser-based regression automation suites and tests and also extend and distribute scripts in many environments. Selenium WebDriver is a collection of language-specific bindings to drive a browser - the way it is meant to bedriven.

Quick Example of Selenium Code
Following is a quick example that uses Chrome Driver to get desired web elements and print out the results from the website https://the-internet.herokuapp.com with Python language.

Tips for Writing Effective Selenium Scripts
Some tips to follow while writing Selenium scripts are as follows:
- To make your tests generic, you should try to pass the initialization values from a configuration file at all times.
- 'wait. Until ()' can help ensure the page is loaded completely.
- To verify if an element exists, you can try to 'get' the web element size. A return value>0 is always a good way.
- Select dropdown options by selecting text, index, or values.
- To switch to a new pop-up window, you can first getAllWindowsHandles() and then switch using the method driver.switchTo()
- If you want to 'hover' the desired web element, better to use the Actions() class to handle it.
- Use the function DeleteAllCookies() of driver.Manager() should you need to clear all the cookies before running your test scripts.
Check out: Selenium Automation Tips You Must Know
Common Problems with Selenium Scripts and How to Solve Them
1. Scalability
The response of web applications in different browsers is not precisely the same. Even if the Selenium web driver allows you to test on multiple versions of browsers or operating systems, testing efficiency may not be ideal due to the limitation of testers and the number of test threads. In addition, Selenium can only run tests in a specific order. Parallel testing can alleviate this problem to some extent.
With HeadSpin, you can run Selenium tests across hundreds of web browsers of different types in parallel.
2. Sequential Steps
When expected events do not occur due to uncertain reasons, the sequence of Web tests may encounter problems. We usually use the waiting defined in Selenium to deal with this problem, such as implicit waiting and explicit waiting.
3. Handling Dynamic Elements
Automated testing of dynamic web applications using Selenium is also often tricky because locators may not interact with web elements. Ajax-based web content loading sometimes takes time, which is also a possible reason for the failure of the test script.
As mentioned earlier, we will use Selenium's weight mechanism, such as implicit wait and explicit wait, or create dynamic or custom XPath to process dynamic web content
4. False-Positive and False-Negative Results
False positives refer to errors in test cases, even if the tested web application usually works. Similarly, false negative means that the test case result passes and the application under test has encountered an error. This will mislead the test team and increase the difficulty of communication between the QA team and the development team. For automated testers, dealing with test instability itself is a challenging task.
To overcome this instability, we need to ensure that test plans, test cases, and test environments are managed strictly, effectively, and organized.
5. Pop-Up and Alert Handling
Different types of pop-up and alerts occur while interacting with a web application, below are a few listed:
- Browser level notification: allow/deny download. Browser level pop-ups need to be explicitly handled according to different browsers. For Chrome browsers, you can use chrome options. For Firefox browsers, you can use Firefox options /Firefox profile.
- Page Alert: i.e., "you will be forwarded to another website," etc. Such alerts can be handled using Selenium's predefined "alerts" class, which provides various methods, such as accept (), deny (), and so on.
- OS level pop-ups: Selenium function is only limited by Web interaction; Unable to process pop-ups at the operating system level.
6. Captcha or OTP
It is challenging for automated test workers to verify whether the user access verification code and OTP content mechanism are used for robot operation.
7. Mobile Testing
People are willing to use mobile devices rather than personal computers to access web applications. There are many mobile devices on the market (different operating systems, versions, screen resolutions, etc.), and it is almost impossible to use Selenium for testing.
With Appium, developers can test content on native mobile operating systems at no harder knowledge level, as Appium also uses the WebDriver protocol and is built from Selenium.
Best Practices for Writing Selenium Scripts
1. Use Proper Locators
Selenium uses pre-built actions to interact with the browser. The types of locators are:
- Class
- ID
- Link Text
- PartPartial Link Textial
- Tag Name
- XPath
Whenever possible, use unique classes or IDs as Selenium locators because they are rarely changed. But locators like link text can change all the time - think of dynamic buttons based on login status or your marketing team running A / B tests. Avoid using XPath navigation whenever possible
2. Browser Zoom Level to be set to 100%
Test scripts may not work correctly on some browsers during Selenium test automation. This is often the case when performing cross-browser tests on outdated browsers such as Internet Explorer.
Along with this setting, Protected Mode Settings (in Internet Explorer) for each zone must be the same; else, you may get a NoSuchWindowException exception.
3. Maximize the Browser Window
To ensure the consistency of the web page layout in the test report. It is recommended to maximize the browser window immediately after loading the test URL to ensure that a screenshot of the entire web page is captured. (Selenium does not open browser windows in maximized mode by default)
4. Use Code Dependency Properly
To make the code more universal and maintainable, it is recommended to build operation methods and dependency files separately to maintain the values to be passed in. Especially for cross-version browser testing.
FAQs
1. What are some of the major changes or upgrades in various versions of Selenium?
- Selenium v1.0
The initial release of Selenium that included three tools: Selenium IDE, Selenium RC, and Selenium Grid
- Selenium v2.0
A Selenium WebDriver was introduced that replaced Selenium RC. With the onset of WebDriver, RC was deprecated and moved to the legacy package.
- Selenium v3.0
Includes new features and functionalities; Selenium IDE, Selenium WebDriver, and Selenium Grid
2. What are some of the programming languages and operating systems supported by Selenium?
- Programming language— C#, Java, Python, PHP, Ruby, Perl, JavaScript
- Operating systems— Android, iOS, Windows, Linux, Mac, Solaris
3. What is XPath in Selenium?
XPath, also known as XML Path, is a language used to query XML documents that assist in locating elements in Selenium. This consists of a path expression along with some conditions. Here, one can easily write XPath script/query to locate any element in the webpage.
4. What are the main types of waits in Selenium?
- Implicit Wait— It defines a period until when the Web Driver should be waiting before throwing the No Such Element exception.
- Explicit Wait— This is used to convey the WebDriver to wait for certain conditions or maximum time exceeded before throwing an 'ElementNotVisibleException' exception. It is an intelligent wait but can only be applied for specific elements.