Mozilla Firefox is one of the most extensively employed web browsers globally. Renowned for its rich feature set, Firefox is well-aligned with many cutting-edge testing methodologies and tools. Among these tools, Selenium takes center stage, leveraging Firefox WebDriver, or GeckoDriver, to seamlessly integrate test cases with the Firefox Browser.
This comprehensive blog delves into the intricacies of running Selenium tests using Firefox WebDriver, providing a detailed example, along with a few code snippets to guide you through the process.
Understanding the Selenium Firefox Driver/GeckoDriver
The Selenium Firefox Driver, also known as GeckoDriver, is a component of Selenium WebDriver specifically designed to automate and control the Firefox web browser during testing. It is a bridge between the Selenium WebDriver scripts and the Firefox browser, facilitating the execution of test cases written in various programming languages.
GeckoDriver is developed by the Mozilla project and is compliant with the W3C WebDriver protocol, enabling seamless communication between the WebDriver client and the Firefox browser. It provides a platform-specific implementation to interact with the browser's rendering engine, allowing testers to perform various actions, such as navigating URLs, clicking elements, filling forms, and validating page content.
The Selenium Firefox Driver, or GeckoDriver, is a crucial tool for automating web testing with Firefox, enabling testers to create robust, reliable, and cross-browser-compatible test suites.
GeckoDriver plays a crucial role in enabling automated testing with the Firefox browser.
GeckoDriver is part of the Selenium WebDriver ecosystem, specifically for automating web browsers using the Firefox browser. Here are several reasons why developers use GeckoDriver:
- Browser Automation: GeckoDriver allows developers and testers to automate interactions with the Firefox browser. This includes navigating to URLs, clicking on elements, filling out forms, and verifying page content.
- Cross-Browser Testing: Selenium WebDriver supports multiple browsers, including Firefox, Chrome, Safari, and Internet Explorer. GeckoDriver enables cross-browser testing by providing the necessary interface to automate Firefox.
- Compatibility: GeckoDriver is designed to work with the latest versions of the Firefox browser, ensuring compatibility and stability for automating web testing tasks.
- WebDriver Protocol: GeckoDriver implements the W3C WebDriver protocol, which defines a standardized way for WebDriver clients to communicate with web browsers. This allows Selenium-based tests to work consistently across different browsers and platforms.
- Open Source: GeckoDriver is an open-source project developed by the Mozilla community. It is freely available for anyone to use, contribute to, and modify according to their needs.
- Community Support: Being part of the Selenium ecosystem, GeckoDriver benefits from a large, active community of developers and testers. This community provides support, documentation, and resources to help users use GeckoDriver for their testing needs.
Overall, GeckoDriver is crucial in enabling automated testing with the Firefox browser, offering a reliable and standardized approach for browser automation within the Selenium framework.
Setting up GeckoDriver for Selenium WebDriver
- Download GeckoDriver: Visit the GeckoDriver releases page on GitHub: GeckoDriver Releases. Download the appropriate version of GeckoDriver for your operating system. Ensure compatibility with your Firefox browser version.
- Extract GeckoDriver: After downloading the GeckoDriver archive, extract the executable file (geckodriver) from the archive and send it to a directory on your system. Make sure to note the path to the directory where you extracted GeckoDriver.
- Set System Property in Selenium Script: In your Selenium script, before creating an instance of the Firefox WebDriver, set the system property "webdriver.gecko.driver" to the path of the GeckoDriver executable. This informs Selenium where to find the GeckoDriver executable on your system.
- Initialize Firefox WebDriver: After setting the system property, create an instance of the Firefox WebDriver in your Selenium script using the FirefoxDriver class.
Replace "/path/to/geckodriver" with your system's actual path to the GeckoDriver executable.
Following these steps, you can set up GeckoDriver for Selenium WebDriver and start automating tests with the Firefox browser.
Read: A Comprehensive Guide to Cross-Platform Mobile Test Automation Using Appium
GeckoDriver Setup for the Latest Selenium Version( 4.21.0)
The latest version of Selenium includes a feature that automatically downloads the required WebDriver binaries for you, including GeckoDriver for Firefox. Here's a detailed explanation of how this works and how you can use it.
1. Automatic Driver Management in the Latest Selenium Version
The latest version of Selenium includes an automatic driver management feature that handles downloading and setting up browser drivers. This means you no longer need to download GeckoDriver and manually specify its path in your code. Selenium will take care of it for you.
2. Updating Selenium:
A Detailed Overview of Selenium Firefox Driver, Selenium Firefox WebDriver, and GeckoDriver
In Selenium, "Selenium Firefox Driver," "Selenium Firefox WebDriver," and "GeckoDriver" are terminologies that are often used interchangeably or confused with each other due to their close relationship. However, they refer to different components within the Selenium ecosystem, specifically for automating interactions with the Firefox browser. Let's clarify each term:
GeckoDriver:
- GeckoDriver is a standalone executable file provided by Mozilla, designed to facilitate communication between Selenium WebDriver scripts and the Firefox browser.
- It acts as a bridge, enabling Selenium WebDriver to control Firefox programmatically.
- GeckoDriver implements the WebDriver protocol and translates WebDriver commands into actions performed by Firefox.
- In simpler terms, GeckoDriver is the actual driver executable responsible for launching and controlling Firefox during automated tests.
Selenium Firefox Driver:
- "Selenium Firefox Driver" typically refers to the WebDriver implementation tailored to the browser.
- This WebDriver implementation is part of the Selenium project and provides the API and functionality needed to interact with Firefox.
- It includes classes and methods for controlling Firefox, such as navigating to URLs, finding elements, interacting with web elements, and managing browser sessions.
Selenium Firefox WebDriver:
- “Selenium Firefox WebDriver" is a broader term encompassing both the GeckoDriver and the Selenium Firefox Driver.
- It refers to combining GeckoDriver and the Selenium WebDriver API specifically used to automate Firefox browser interactions.
- GeckoDriver and the Selenium Firefox Driver enable testers and developers to automate Firefox browser testing using Selenium WebDriver.
GeckoDriver is the executable responsible for launching and controlling Firefox, while the Selenium Firefox Driver provides the API and functionality to interact with Firefox programmatically. Together, they form the Selenium Firefox WebDriver, enabling automated testing of web applications in the Firefox browser using Selenium.
How to run in the HeadSpin Platform?
You can obtain the capabilities and WebDriver URL by clicking the 'More Actions' button (the three dots on the far right side of each device).
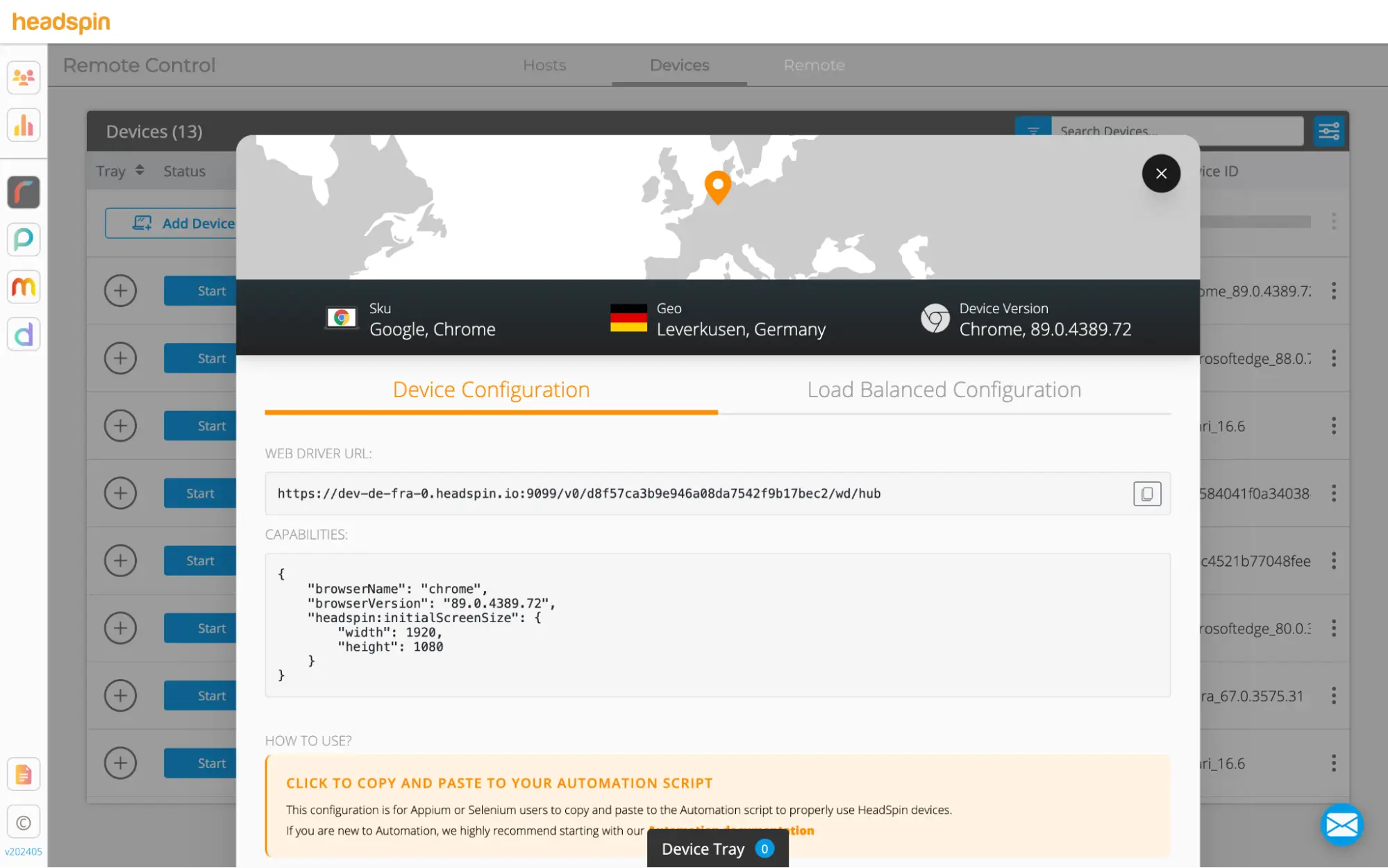
Enhancing Testing Capabilities with HeadSpin
HeadSpin is a powerful platform that provides a comprehensive solution for testing the performance of apps. It offers unique features and support for Selenium testing on Firefox, making it an invaluable tool for testers and developers.
Key Benefits of Using HeadSpin
- Real Device Testing: HeadSpin allows you to run Selenium tests on real devices, providing more accurate and reliable test results than emulators or simulators.
- Global Device Infrastructure: With HeadSpin, you can test your applications on devices in various regions worldwide, ensuring your app performs well in different network conditions and environments.
- Performance Monitoring: HeadSpin provides detailed performance metrics and insights, helping you identify and fix performance bottlenecks in your application.
- Continuous Testing: Integrate HeadSpin with your CI/CD pipeline to enable continuous testing and ensure your application is always functioning optimally.
- Scalability: HeadSpin's infrastructure can scale to meet your testing needs, whether running a few or thousands of concurrent tests.
Final Thoughts
GeckoDriver is crucial in enabling automated testing with the Firefox browser, offering a reliable and standardized approach for browser automation within the Selenium framework. By setting up GeckoDriver correctly, you can efficiently automate your Firefox browser tests.
Additionally, leveraging the capabilities of HeadSpin can significantly enhance your testing process, providing real device testing, performance monitoring, and global test coverage. Start optimizing your Selenium tests with Firefox WebDriver today, and take advantage of HeadSpin's robust features to ensure your applications perform flawlessly.
FAQs
Q1. Can I use GeckoDriver with other programming languages besides Python?
Ans: Yes, GeckoDriver can be used with various programming languages Selenium supports, such as Java, C#, Ruby, and JavaScript. Each language will have Selenium bindings and methods to interact with GeckoDriver.
Q2. How do I update GeckoDriver to the latest version?
Ans: The GeckoDriver releases page on GitHub will have the latest version for your OS. Replace the old GeckoDriver executable with the new one in your system’s directory, and ensure your Selenium scripts point to the updated path.
Q3. What should I do if GeckoDriver is not working with the latest Firefox update?
Ans: If GeckoDriver isn't working with the latest Firefox update, ensure that you have the latest version of GeckoDriver installed. You can experience compatibility issues if the driver version is outdated. Additionally, check the Selenium and Firefox release notes for any known issues or required changes.